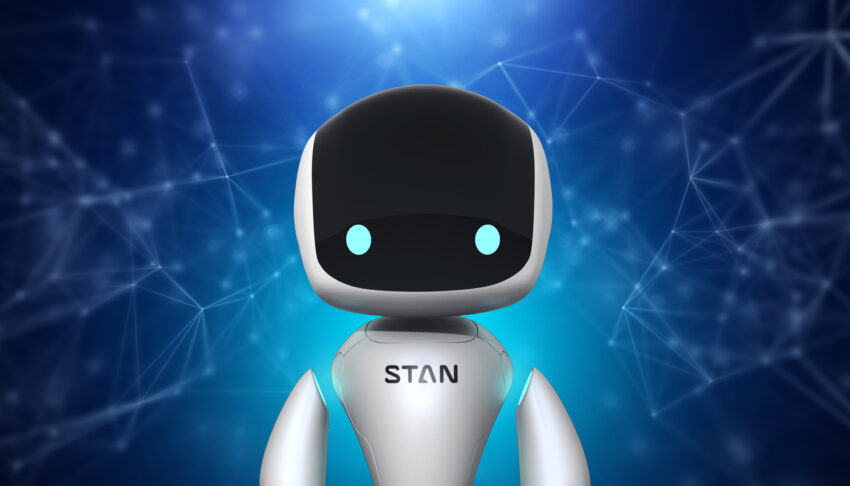
05 Apr How to get a stack trace from an error in JavaScript
As a JavaScript developer, you know that errors can occur in unexpected places and that it’s important to be able to debug them. In this tutorial, we’ll learn how to do exactly that: get the stack trace of an error so you can easily find out what happened! The content is brought to you by https://technochatnews.com/
How to get a stack trace from an error in JavaScript
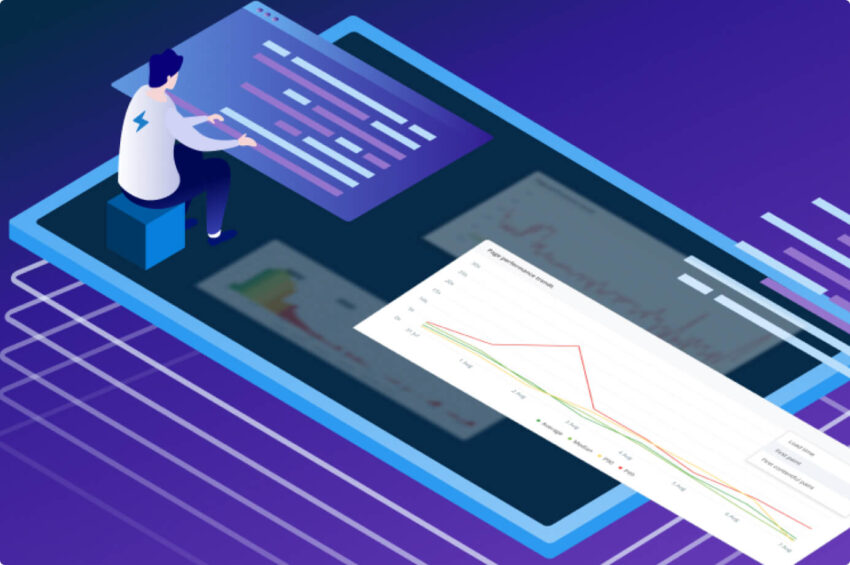
Image source: Google.com
A stack trace is a list of functions that were executed before an error occurred. It can help you determine where an error is coming from and make it easier to debug your code. Keep reading: See Your Page and Post Views on WordPress
To get the current call stack in JavaScript, use the debugger statement:
Finding the line of code that generated the error
You can use the debugger to find the line of code that generated the error.
To do this, add a debugger statement to your JavaScript file and run it in Chrome:
“`javascript
debugger;
“`
You’ll see something like this:
The stack trace indicates that there is an error at line 8 of your script. In this example, we’ve used console.log() to print out “I’m on line 8!” because we want you to see how useful console logging can be when debugging problems with your code!
Finding to which function the error originated
The next step is to find the function that generated the error. In order to do this, you’ll need to trace your way back through all of the functions that have been called before reaching the one that threw an exception.
To do so:
- Open your browser’s debugger (Google Chrome has a built-in debugger).
- Set breakpoints at each level of the call until you reach where your code is being executed (this may be in another file).
Using the browser’s debugger and console.log() method
To get more information about an error in JavaScript, you can use the debugger statement. This will allow you to break into your code at any time and explore what’s going on inside it.
To do this in Chrome, open up a new file and add some code that generates an error:
console.log(“hello”); // TypeError: Cannot read property ‘name’ of undefined; console logs undefined as well as hello
In this case, we’re trying to access the name property on an undeclared variable (console). When we run it in our browser window with F12 DevTools open, we’ll see something like this:
TypeError: Cannot read property ‘name’ of undefined. The error message gives us a hint as to where things went wrong – there is no name property defined yet! Let’s change our script so it looks like this instead: var greeting = “Hello”; var person = {}; Person instances have names associated with them.console.log(person[greeting]); Now when we run it again…
Conclusion
We hope that we have been able to help you understand how to get a stack trace from an error in JavaScript.
No Comments